In order to memorize methods faster (we want to focus on the result and not get into the habit of constantly referring to manual, right?), I will explain what I was thinking while making them. But first take a look at this tree:
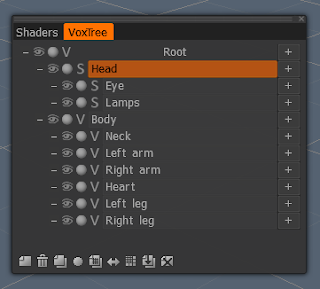
In the first post I promised to be concise. So, the code itself:
void main() {
// preparing a scene
Vox v;
v.clearScene();
// building a tree with v-layers
v
.appendToParent( "Head" )
.appendToParent( "Body" )
.append( "Neck" )
.appendToParent( "Left arm" )
.appendToParent( "Right arm" )
.appendToParent( "Heart" )
.appendToParent( "Left leg" )
.appendToParent( "Right leg" )
// return to the layer "Head", switch it
// to "Surface mode" and attach
.to( "Head" ).toSurface()
.append( "Eye" )
.appendToParent( "Lamps" )
;
// removing a first layer from a scene
v.firstForRoot().remove();
}
Do you see patterns of methods? `append()`, `appendToParent()`, `appendToRoot()`, `forEach()`, `forRootEach()`, ... - as you might have noticed, the names themselves are pretty self-explanatory. Compare the code with the resulting picture. It should be easy to figure out without the need to frequently refer to documentation, which command does what.
After looking through the libraries for working with 'trees' I found that it is most intuitively done in JQuery. Are you familiar with it? Then I won't explain anything!) The only thing that should be taken into account during developing: class `Vox` works with 3D-Coat's UI, so introduced in code Vox-methods select layers before any kind of operation.
So, working on layers:
void main() {
// creating a tree - see above
// ...
// #1
// calling invert() for all v-layer
// see below
v.forRootEach( "invert" );
// #2
// moving to the "Body" and invert() for it and childs
v.to( "Body" ).call( "invert" ).forEach( "invert" );
}
void invert() {
Vox v;
v.isSurface() ? v.toVoxel() : v.toSurface();
Wait( 50 );
}
I think that previously described methods are enough for processing any tree. But Andrew Shpagin says it is not. Here you have another variant:
// access by index on all childs of "Head"
v.to( "Head" ).call( "invert" );
for ( int i = 0; i < v.count(); ++i ) {
v.at( i ).call( "invert" ).parent();
Wait( 100 );
}
Also, all VoxTree options are available for 'Vox' class via scripts:

What I also would like to add is a more concise constructor. Later. I need to improve operations with primitives, and there are a lot of them...
P.S. Btw, the example tree has a "real prototype":
Комментариев нет:
Отправить комментарий